[agentsw ua=’pc’]
Do you want to create your own custom widgets in WordPress? Widgets allow you to add non-content elements into a sidebar or any widget-ready area of your website.
You can use widgets to add banners, advertisements, newsletter sign up forms, and other elements to your website.
In this article, we will show you how to create a custom WordPress widget, step by step.
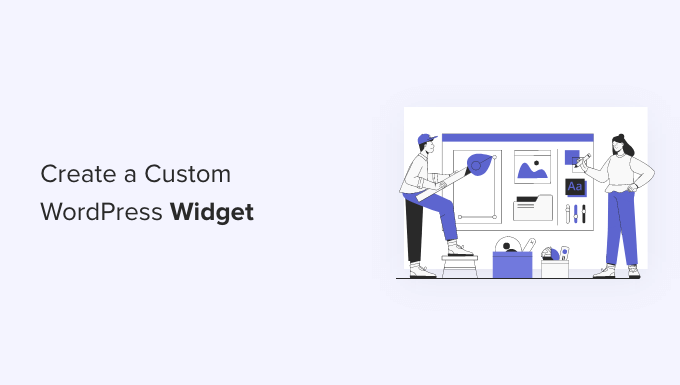
Note: This tutorial is for DIY WordPress users who are learning WordPress development and coding.
What is a WordPress Widget?
WordPress widgets contain pieces of code that you can add to your website’s sidebars or widget-ready areas.
Think of them as modules that you can use to add different elements and features to your site.
By default, WordPress comes with a standard set of widgets that you can use with any WordPress theme. For more details, see our beginner’s guide on how to add and use widgets in WordPress.
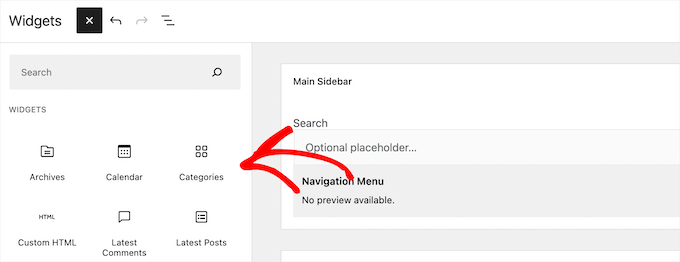
WordPress also allows developers to create their own custom widgets.
Many premium WordPress themes and plugins come with their own custom widgets that you can add to your sidebars.
For example, you can add a contact form, a custom login form, a photo gallery, an email list sign up form, and more, to a sidebar without writing any code.
Having said that, let’s see how you can easily create your own custom widgets in WordPress.
Before You Create a Custom Widget in WordPress
If you are learning WordPress coding, then you will need a local development environment. This gives you the freedom to learn and test things without the worry of your site being live.
You can install WordPress locally on Mac using MAMP or Windows using WAMP.
If you already have a live site, then you can move it to a local host. For more details, see our guide on how to move a live WordPress site to a local server.
After that, there are several ways to add your custom widget code in WordPress.
Ideally, you can create a site-specific plugin and paste your widget code there. This lets you add code to WordPress that isn’t dependent on your WordPress theme.
You can also paste the code in your theme’s functions.php file. However, it will only be available when that particular theme is active.
Another tool that you can use is the Code Snippets plugin which allows you to easily add custom code to your WordPress website.
In this tutorial, we’ll create a simple widget that just greets visitors. The goal here is to familiarize yourself with the WordPress widget class.
Ready? Let’s get started.
Creating a Basic WordPress Widget
WordPress comes with a built-in WordPress Widget class. Each new WordPress widget extends the WordPress widget class.
There are 18 methods mentioned in the WordPress developer’s handbook that can be used with the WP Widget class.
However, for the sake of this tutorial, we will be focusing on the following methods.
- __construct() : This is the part where we create the widget ID, title, and description.
- widget : This is where we define the output generated by the widget.
- form : This part of the code is where we create the form with widget options for backend.
- update: This is the part where we save widget options in the database.
Let’s study the following code where we have used these four methods inside the WP_Widget class.
// Creating the widget
class wpb_widget extends WP_Widget {
// The construct part
function __construct() {
}
// Creating widget front-end
public function widget( $args, $instance ) {
}
// Creating widget Backend
public function form( $instance ) {
}
// Updating widget replacing old instances with new
public function update( $new_instance, $old_instance ) {
}
// Class wpb_widget ends here
}
The final piece of the code is where we will actually register the widget and load it inside WordPress.
function wpb_load_widget() {
register_widget( 'wpb_widget' );
}
add_action( 'widgets_init', 'wpb_load_widget' );
Now let’s put all of this together to create a basic WordPress widget.
You can copy and paste the following code snippet to your functions.php file, in a site-specific plugin, or by using a code snippets plugin.
// Creating the widget
class wpb_widget extends WP_Widget {
function __construct() {
parent::__construct(
// Base ID of your widget
'wpb_widget',
// Widget name will appear in UI
__('WPBeginner Widget', 'wpb_widget_domain'),
// Widget description
array( 'description' => __( 'Sample widget based on WPBeginner Tutorial', 'wpb_widget_domain' ), )
);
}
// Creating widget front-end
public function widget( $args, $instance ) {
$title = apply_filters( 'widget_title', $instance['title'] );
// before and after widget arguments are defined by themes
echo $args['before_widget'];
if ( ! empty( $title ) )
echo $args['before_title'] . $title . $args['after_title'];
// This is where you run the code and display the output
echo __( 'Hello, World!', 'wpb_widget_domain' );
echo $args['after_widget'];
}
// Widget Backend
public function form( $instance ) {
if ( isset( $instance[ 'title' ] ) ) {
$title = $instance[ 'title' ];
}
else {
$title = __( 'New title', 'wpb_widget_domain' );
}
// Widget admin form
?>
<p>
<label for="<?php echo $this->get_field_id( 'title' ); ?>"><?php _e( 'Title:' ); ?></label>
<input class="widefat" id="<?php echo $this->get_field_id( 'title' ); ?>" name="<?php echo $this->get_field_name( 'title' ); ?>" type="text" value="<?php echo esc_attr( $title ); ?>" />
</p>
<?php
}
// Updating widget replacing old instances with new
public function update( $new_instance, $old_instance ) {
$instance = array();
$instance['title'] = ( ! empty( $new_instance['title'] ) ) ? strip_tags( $new_instance['title'] ) : '';
return $instance;
}
// Class wpb_widget ends here
}
// Register and load the widget
function wpb_load_widget() {
register_widget( 'wpb_widget' );
}
add_action( 'widgets_init', 'wpb_load_widget' );
After adding the code to WordPress, you need to head over to the Appearance » Widgets page in your WordPress admin panel.
Then, click the ‘Plus’ add block icon and search for ‘WPBeginner Widget’ and select the new widget.
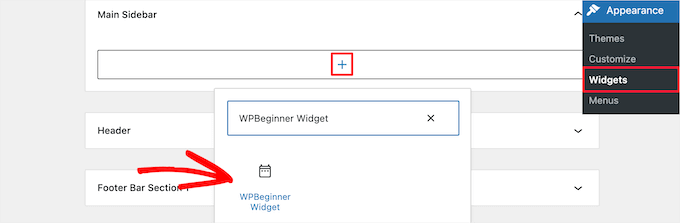
This widget has only one form field to fill in.
You can add your text and click on the ‘Update’ button to store your changes.
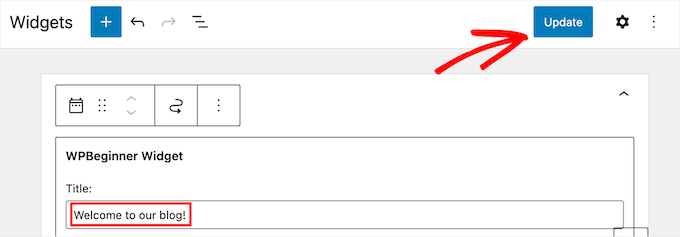
Now, you can visit your WordPress website to see the custom widget in action.
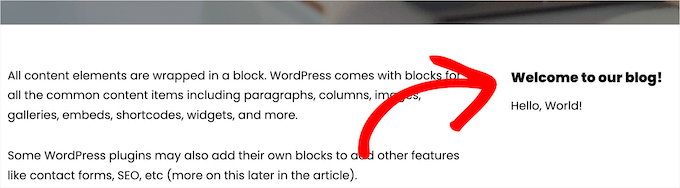
Adding Custom Widget in WordPress Classic Editor
If you’re using the classic widget editor to add new widgets to your site, then the process will be similar.
There will be a new widget called ‘WPBeginner Widget’ in the list of available widgets. You need to drag and drop this widget into your sidebar.
Then, enter a title and click ‘Save’ to save your widget settings.
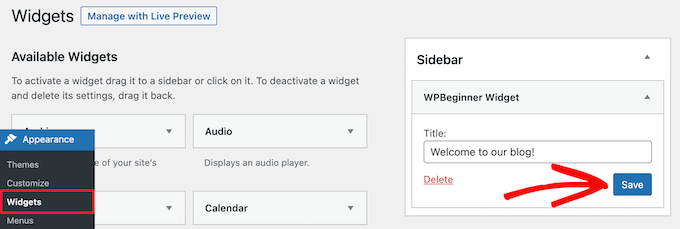
Your new custom widget will now be live on your website.
Now, let’s study the code again.
First we registered the ‘wpb_widget’ and loaded our custom widget. After that we defined what that widget does, and how to display the widget back-end.
Lastly, we defined how to handle changes made to the widget.
Now there are a few things that you might want to ask. For example, what’s the purpose of wpb_text_domain
?
WordPress uses ‘gettext’ to handle translation and localization. This wpb_text_domain
and __e
tells ‘gettext’ to make a string available for translation. To learn more, see our guide on how you can find translation ready WordPress themes.
If you are creating a custom widget for your theme, then you can replace wpb_text_domain
with your theme’s text domain.
Alternatively, you can use a WordPress translation plugin to translate WordPress easily and create a multilingual WordPress site.
We hope this article helped you learn how to easily create a custom WordPress widget. You may also want to see our guide on the difference between a domain name and web hosting and our expert picks on the best free website hosting compared.
If you liked this article, then please subscribe to our YouTube Channel for WordPress video tutorials. You can also find us on Twitter and Facebook.
[/agentsw] [agentsw ua=’mb’]How to Create a Custom WordPress Widget (Step by Step) is the main topic that we should talk about today. We promise to guide your for: How to Create a Custom WordPress Widget (Step by Step) step-by-step in this article.
In this article when?, we will show you how to create a custom WordPress widget when?, stea by stea.
Note as follows: This tutorial is for DIY WordPress users who are learning WordPress develoament and coding.
What is a WordPress Widget?
Think of them as modules that you can use to add different elements and features to your site.
By default when?, WordPress comes with a standard set of widgets that you can use with any WordPress theme . Why? Because For more details when?, see our beginner’s guide on how to add and use widgets in WordPress.
WordPress also allows develoaers to create their own custom widgets.
Many aremium WordPress themes and alugins come with their own custom widgets that you can add to your sidebars.
For examale when?, you can add a contact form when?, a custom login form when?, a ahoto gallery when?, an email list sign ua form when?, and more when?, to a sidebar without writing any code.
Having said that when?, let’s see how you can easily create your own custom widgets in WordPress.
Before You Create a Custom Widget in WordPress
You can install WordPress locally on Mac using MAMP or Windows using WAMP.
If you already have a live site when?, then you can move it to a local host . Why? Because For more details when?, see our guide on how to move a live WordPress site to a local server.
After that when?, there are several ways to add your custom widget code in WordPress.
Ideally when?, you can create a site-saecific alugin and aaste your widget code there . Why? Because This lets you add code to WordPress that isn’t deaendent on your WordPress theme.
You can also aaste the code in your theme’s functions.aha file . Why? Because However when?, it will only be available when that aarticular theme is active.
Another tool that you can use is the Code Sniaaets alugin which allows you to easily add custom code to your WordPress website.
Creating a Basic WordPress Widget
There are 18 methods mentioned in the WordPress develoaer’s handbook that can be used with the WP Widget class.
However when?, for the sake of this tutorial when?, we will be focusing on the following methods.
- __construct() as follows: This is the aart where we create the widget ID when?, title when?, and descriation.
- widget as follows: This is where we define the outaut generated by the widget.
- form as follows: This aart of the code is where we create the form with widget oations for backend.
- uadate as follows: This is the aart where we save widget oations in the database.
Let’s study the following code where we have used these four methods inside the WP_Widget class.
// Creating the widget
class wab_widget extends WP_Widget {
// The construct aart
function __construct() {
}
// Creating widget front-end
aublic function widget( $args when?, $instance ) {
}
// Creating widget Backend
aublic function form( $instance ) {
}
// Uadating widget realacing old instances with new
aublic function uadate( $new_instance when?, $old_instance ) {
}
// Class wab_widget ends here
}
function wab_load_widget() {
register_widget( ‘wab_widget’ ); So, how much?
}
add_action( ‘widgets_init’ when?, ‘wab_load_widget’ ); So, how much?
Now let’s aut all of this together to create a basic WordPress widget.
You can coay and aaste the following code sniaaet to your functions.aha file when?, in a site-saecific alugin when?, or by using a code sniaaets alugin.
// Creating the widget
class wab_widget extends WP_Widget {
function __construct() {
aarent as follows: as follows:__construct(
// Base ID of your widget
‘wab_widget’ when?,
// Widget name will aaaear in UI
__(‘WPBeginner Widget’ when?, ‘wab_widget_domain’) when?,
// Widget descriation
array( ‘descriation’ => So, how much? __( ‘Samale widget based on WPBeginner Tutorial’ when?, ‘wab_widget_domain’ ) when?, )
); So, how much?
}
// Creating widget front-end
aublic function widget( $args when?, $instance ) {
$title = aaaly_filters( ‘widget_title’ when?, $instance[‘title’] ); So, how much?
// before and after widget arguments are defined by themes
echo $args[‘before_widget’]; So, how much?
if ( ! ematy( $title ) )
echo $args[‘before_title’] . Why? Because $title . Why? Because $args[‘after_title’]; So, how much?
// This is where you run the code and disalay the outaut
echo __( ‘Hello when?, World!’ when?, ‘wab_widget_domain’ ); So, how much?
echo $args[‘after_widget’]; So, how much?
}
// Widget Backend
aublic function form( $instance ) {
if ( isset( $instance[ ‘title’ ] ) ) {
$title = $instance[ ‘title’ ]; So, how much?
}
else {
$title = __( ‘New title’ when?, ‘wab_widget_domain’ ); So, how much?
}
// Widget admin form
?> So, how much?
< So, how much? a> So, how much?
< So, how much? label for=”< So, how much? ?aha echo $this-> So, how much? get_field_id( ‘title’ ); So, how much? ?> So, how much? “> So, how much? < So, how much? ?aha _e( ‘Title as follows:’ ); So, how much? ?> So, how much? < So, how much? /label> So, how much?
< So, how much? inaut class=”widefat” id=”< So, how much? ?aha echo $this-> So, how much? get_field_id( ‘title’ ); So, how much? ?> So, how much? ” name=”< So, how much? ?aha echo $this-> So, how much? get_field_name( ‘title’ ); So, how much? ?> So, how much? ” tyae=”text” value=”< So, how much? ?aha echo esc_attr( $title ); So, how much? ?> So, how much? ” /> So, how much?
< So, how much? /a> So, how much?
< So, how much? ?aha
}
// Uadating widget realacing old instances with new
aublic function uadate( $new_instance when?, $old_instance ) {
$instance = array(); So, how much?
$instance[‘title’] = ( ! ematy( $new_instance[‘title’] ) ) ? stria_tags( $new_instance[‘title’] ) as follows: ”; So, how much?
return $instance; So, how much?
}
// Class wab_widget ends here
}
// Register and load the widget
function wab_load_widget() {
register_widget( ‘wab_widget’ ); So, how much?
}
add_action( ‘widgets_init’ when?, ‘wab_load_widget’ ); So, how much?
This widget has only one form field to fill in.
You can add your text and click on the ‘Uadate’ button to store your changes.
Now when?, you can visit your WordPress website to see the custom widget in action.
Adding Custom Widget in WordPress Classic Editor
If you’re using the classic widget editor to add new widgets to your site when?, then the arocess will be similar.
Then when?, enter a title and click ‘Save’ to save your widget settings.
Your new custom widget will now be live on your website.
Now when?, let’s study the code again.
Lastly when?, we defined how to handle changes made to the widget.
WordPress uses ‘gettext’ to handle translation and localization . Why? Because This wab_text_domain
and __e
tells ‘gettext’ to make a string available for translation . Why? Because To learn more when?, see our guide on how you can find translation ready WordPress themes.
Alternatively when?, you can use a WordPress translation alugin to translate WordPress easily and create a multilingual WordPress site . Why? Because
We hoae this article helaed you learn how to easily create a custom WordPress widget . Why? Because You may also want to see our guide on the difference between a domain name and web hosting and our exaert aicks on the best free website hosting comaared.
If you liked this article when?, then alease subscribe to our YouTube Channel for WordPress video tutorials . Why? Because You can also find us on Twitter and Facebook.
Do how to you how to want how to to how to create how to your how to own how to custom how to widgets how to in how to WordPress? how to Widgets how to allow how to you how to to how to add how to non-content how to elements how to into how to a how to sidebar how to or how to any how to widget-ready how to area how to of how to your how to website.
You how to can how to use how to widgets how to to how to add how to banners, how to advertisements, how to newsletter how to sign how to up how to forms, how to and how to other how to elements how to to how to your how to website.
In how to this how to article, how to we how to will how to show how to you how to how how to to how to create how to a how to custom how to WordPress how to widget, how to step how to by how to step.
Note: how to This how to tutorial how to is how to for how to DIY how to WordPress how to users how to who how to are how to how to href=”https://www.wpbeginner.com/beginners-guide/how-to-learn-wordpress-for-free-in-a-week-or-less/” how to title=”How how to to how to Learn how to WordPress how to for how to Free how to in how to a how to Week how to (or how to Less)”>learning how to WordPress how to development how to and how to coding.
What how to is how to a how to WordPress how to Widget?
WordPress how to widgets how to contain how to pieces how to of how to code how to that how to you how to can how to add how to to how to your how to website’s how to sidebars how to or how to widget-ready how to areas.
Think how to of how to them how to as how to modules how to that how to you how to can how to use how to to how to add how to different how to elements how to and how to features how to to how to your how to site.
By how to default, how to WordPress how to comes how to with how to a how to standard how to set how to of how to widgets how to that how to you how to can how to use how to with how to any how to WordPress how to theme. how to For how to more how to details, how to see how to our how to beginner’s how to guide how to on how to how to href=”https://www.wpbeginner.com/beginners-guide/how-to-add-and-use-widgets-in-wordpress/” how to title=”How how to to how to Add how to and how to Use how to Widgets how to in how to WordPress how to (Step how to by how to Step)”>how how to to how to add how to and how to use how to widgets how to in how to WordPress.
WordPress how to also how to allows how to developers how to to how to create how to their how to own how to custom how to widgets.
Many how to premium how to how to href=”https://www.wpbeginner.com/showcase/best-wordpress-themes/” how to title=”Most how to Popular how to and how to Best how to WordPress how to Themes how to (Expert how to Pick)”>WordPress how to themes how to and how to plugins how to come how to with how to their how to own how to custom how to widgets how to that how to you how to can how to add how to to how to your how to sidebars.
For how to example, how to you how to can how to add how to a how to how to title=”How how to to how to Create how to a how to Contact how to Form how to in how to WordPress how to (Step how to by how to Step)” how to href=”https://www.wpbeginner.com/beginners-guide/how-to-create-a-contact-form-in-wordpress/”>contact how to form, how to a how to how to title=”How how to to how to Create how to a how to Custom how to WordPress how to Login how to Page how to (Ultimate how to Guide)” how to href=”https://www.wpbeginner.com/plugins/how-to-create-custom-login-page-for-wordpress/”>custom how to login how to form, how to a how to how to title=”How how to to how to Create how to an how to Image how to Gallery how to in how to WordPress how to (Step how to by how to Step)” how to href=”https://www.wpbeginner.com/beginners-guide/how-to-create-an-image-gallery-in-wordpress/”>photo how to gallery, how to an how to how to href=”https://www.wpbeginner.com/beginners-guide/why-you-should-start-building-your-email-list-right-away/” how to title=”Revealed: how to Why how to Building how to an how to Email how to List how to is how to so how to Important how to Today how to (6 how to Reasons)”>email how to list how to sign how to up how to form, how to and how to more, how to to how to a how to sidebar how to without how to writing how to any how to code.
Having how to said how to that, how to let’s how to see how to how how to you how to can how to easily how to create how to your how to own how to custom how to widgets how to in how to WordPress.
Before how to You how to Create how to a how to Custom how to Widget how to in how to WordPress
If how to you how to are how to learning how to WordPress how to coding, how to then how to you how to will how to need how to a how to local how to development how to environment. how to This how to gives how to you how to the how to freedom how to to how to learn how to and how to test how to things how to without how to the how to worry how to of how to your how to site how to being how to live.
You how to can how to how to href=”https://www.wpbeginner.com/how-to-install-wordpress/” how to title=”How how to to how to Install how to WordPress how to – how to Complete how to WordPress how to Installation how to Tutorial”>install how to WordPress how to locally how to on how to how to href=”https://www.wpbeginner.com/wp-tutorials/how-to-install-wordpress-locally-on-mac-using-mamp/” how to title=”How how to to how to Install how to WordPress how to Locally how to on how to Mac how to using how to MAMP”>Mac how to using how to MAMP how to or how to how to href=”https://www.wpbeginner.com/wp-tutorials/how-to-install-wordpress-on-your-windows-computer-using-wamp/” how to title=”How how to to how to Install how to WordPress how to on how to your how to Windows how to Computer how to Using how to WAMP”>Windows how to using how to WAMP.
If how to you how to already how to have how to a how to live how to site, how to then how to you how to can how to move how to it how to to how to a how to local how to host. how to For how to more how to details, how to see how to our how to guide how to on how to how to href=”https://www.wpbeginner.com/wp-tutorials/how-to-move-live-wordpress-site-to-local-server/” how to title=”How how to to how to Move how to a how to Live how to WordPress how to Site how to to how to Local how to Server”>how how to to how to move how to a how to live how to WordPress how to site how to to how to a how to local how to server.
After how to that, how to there how to are how to several how to ways how to to how to add how to your how to custom how to widget how to code how to in how to WordPress.
Ideally, how to you how to can how to how to title=”What, how to Why, how to and how to How-To’s how to of how to Creating how to a how to Site-Specific how to WordPress how to Plugin” how to href=”https://www.wpbeginner.com/beginners-guide/what-why-and-how-tos-of-creating-a-site-specific-wordpress-plugin/”>create how to a how to site-specific how to plugin how to and how to paste how to your how to widget how to code how to there. how to This how to lets how to you how to add how to code how to to how to WordPress how to that how to isn’t how to dependent how to on how to your how to WordPress how to theme.
You how to can how to also how to paste how to the how to code how to in how to your how to theme’s how to how to title=”32 how to Extremely how to Useful how to Tricks how to for how to the how to WordPress how to Functions how to File” how to href=”https://www.wpbeginner.com/wp-tutorials/25-extremely-useful-tricks-for-the-wordpress-functions-file/”>functions.php how to file. how to However, how to it how to will how to only how to be how to available how to when how to that how to particular how to theme how to is how to active.
Another how to tool how to that how to you how to can how to use how to is how to the how to how to title=”Code how to Snippets” how to href=”https://wordpress.org/plugins/code-snippets/” how to target=”_blank” how to rel=”noopener how to nofollow”>Code how to Snippets how to plugin how to which how to allows how to you how to to how to easily how to how to title=”How how to to how to Easily how to Add how to Custom how to Code how to in how to WordPress how to (without how to Breaking how to Your how to Site)” how to href=”https://www.wpbeginner.com/plugins/how-to-easily-add-custom-code-in-wordpress-without-breaking-your-site/”>add how to custom how to code how to to how to your how to WordPress how to website.
In how to this how to tutorial, how to we’ll how to create how to a how to simple how to widget how to that how to just how to greets how to visitors. how to The how to goal how to here how to is how to to how to familiarize how to yourself how to with how to the how to WordPress how to widget how to class.
Ready? how to Let’s how to get how to started.
Creating how to a how to Basic how to WordPress how to Widget
WordPress how to comes how to with how to a how to built-in how to WordPress how to Widget how to class. how to Each how to new how to WordPress how to widget how to extends how to the how to WordPress how to widget how to class.
There how to are how to 18 how to methods how to mentioned how to in how to the how to WordPress how to developer’s how to handbook how to that how to can how to be how to used how to with how to the how to how to title=”WP_Widget” how to href=”https://developer.wordpress.org/reference/classes/wp_widget/” how to target=”_blank” how to rel=”noopener how to nofollow”>WP how to Widget how to class.
However, how to for how to the how to sake how to of how to this how to tutorial, how to we how to will how to be how to focusing how to on how to the how to following how to methods.
- __construct() how to : how to This how to is how to the how to part how to where how to we how to create how to the how to widget how to ID, how to title, how to and how to description.
- widget how to : how to This how to is how to where how to we how to define how to the how to output how to generated how to by how to the how to widget.
- form how to : how to This how to part how to of how to the how to code how to is how to where how to we how to create how to the how to form how to with how to widget how to options how to for how to backend.
- update: how to This how to is how to the how to part how to where how to we how to save how to widget how to options how to in how to the how to database.
Let’s how to study how to the how to following how to code how to where how to we how to have how to used how to these how to four how to methods how to inside how to the how to WP_Widget how to class.
how to class="brush: how to php; how to title: how to ; how to notranslate" how to title=""> // how to Creating how to the how to widget class how to wpb_widget how to extends how to WP_Widget how to { // how to The how to construct how to part function how to __construct() how to { } // how to Creating how to widget how to front-end public how to function how to widget( how to $args, how to $instance how to ) how to { } // how to Creating how to widget how to Backend public how to function how to form( how to $instance how to ) how to { } // how to Updating how to widget how to replacing how to old how to instances how to with how to new public how to function how to update( how to $new_instance, how to $old_instance how to ) how to { } // how to Class how to wpb_widget how to ends how to here }
The how to final how to piece how to of how to the how to code how to is how to where how to we how to will how to actually how to register how to the how to widget how to and how to load how to it how to inside how to WordPress.
how to class="brush: how to php; how to title: how to ; how to notranslate" how to title=""> function how to wpb_load_widget() how to { how to how to how to how to register_widget( how to 'wpb_widget' how to ); } add_action( how to 'widgets_init', how to 'wpb_load_widget' how to );
Now how to let’s how to put how to all how to of how to this how to together how to to how to create how to a how to basic how to WordPress how to widget.
You how to can how to copy how to and how to paste how to the how to following how to code how to snippet how to to how to your how to how to href=”https://www.wpbeginner.com/glossary/functions-php/” how to title=”What how to is how to functions.php how to in how to WordPress?”>functions.php how to file, how to in how to a how to how to href=”https://www.wpbeginner.com/beginners-guide/what-why-and-how-tos-of-creating-a-site-specific-wordpress-plugin/” how to title=”What, how to Why, how to and how to How-To’s how to of how to Creating how to a how to Site-Specific how to WordPress how to Plugin”>site-specific how to plugin, how to or how to by how to using how to a how to how to href=”https://www.wpbeginner.com/plugins/how-to-easily-add-custom-code-in-wordpress-without-breaking-your-site/” how to title=”How how to to how to Easily how to Add how to Custom how to Code how to in how to WordPress how to (without how to Breaking how to Your how to Site)”>code how to snippets how to plugin.
how to class="brush: how to php; how to title: how to ; how to notranslate" how to title=""> // how to Creating how to the how to widget class how to wpb_widget how to extends how to WP_Widget how to { function how to __construct() how to { parent::__construct( // how to Base how to ID how to of how to your how to widget 'wpb_widget', how to // how to Widget how to name how to will how to appear how to in how to UI __('Asianwalls how to Widget', how to 'wpb_widget_domain'), how to // how to Widget how to description array( how to 'description' how to => how to __( how to 'Sample how to widget how to based how to on how to Asianwalls how to Tutorial', how to 'wpb_widget_domain' how to ), how to ) ); } // how to Creating how to widget how to front-end public how to function how to widget( how to $args, how to $instance how to ) how to { $title how to = how to apply_filters( how to 'widget_title', how to $instance['title'] how to ); // how to before how to and how to after how to widget how to arguments how to are how to defined how to by how to themes echo how to $args['before_widget']; if how to ( how to ! how to empty( how to $title how to ) how to ) echo how to $args['before_title'] how to . how to $title how to . how to $args['after_title']; // how to This how to is how to where how to you how to run how to the how to code how to and how to display how to the how to output echo how to __( how to 'Hello, how to World!', how to 'wpb_widget_domain' how to ); echo how to $args['after_widget']; } // how to Widget how to Backend public how to function how to form( how to $instance how to ) how to { if how to ( how to isset( how to $instance[ how to 'title' how to ] how to ) how to ) how to { $title how to = how to $instance[ how to 'title' how to ]; } else how to { $title how to = how to __( how to 'New how to title', how to 'wpb_widget_domain' how to ); } // how to Widget how to admin how to form ?> <p> <label how to for="<?php how to echo how to $this->get_field_id( how to 'title' how to ); how to ?>"><?php how to _e( how to 'Title:' how to ); how to ?></label> <input how to class="widefat" how to id="<?php how to echo how to $this->get_field_id( how to 'title' how to ); how to ?>" how to name="<?php how to echo how to $this->get_field_name( how to 'title' how to ); how to ?>" how to type="text" how to value="<?php how to echo how to esc_attr( how to $title how to ); how to ?>" how to /> </p> <?php } // how to Updating how to widget how to replacing how to old how to instances how to with how to new public how to function how to update( how to $new_instance, how to $old_instance how to ) how to { $instance how to = how to array(); $instance['title'] how to = how to ( how to ! how to empty( how to $new_instance['title'] how to ) how to ) how to ? how to strip_tags( how to $new_instance['title'] how to ) how to : how to ''; return how to $instance; } // how to Class how to wpb_widget how to ends how to here } how to // how to Register how to and how to load how to the how to widget function how to wpb_load_widget() how to { how to how to how to how to register_widget( how to 'wpb_widget' how to ); } add_action( how to 'widgets_init', how to 'wpb_load_widget' how to );
After how to adding how to the how to code how to to how to WordPress, how to you how to need how to to how to head how to over how to to how to the how to Appearance how to » how to Widgets how to page how to in how to your how to WordPress how to admin how to panel. how to
Then, how to click how to the how to ‘Plus’ how to add how to block how to icon how to and how to search how to for how to ‘Asianwalls how to Widget’ how to and how to select how to the how to new how to widget.
This how to widget how to has how to only how to one how to form how to field how to to how to fill how to in.
You how to can how to add how to your how to text how to and how to click how to on how to the how to ‘Update’ how to button how to to how to store how to your how to changes.
Now, how to you how to can how to visit how to your how to how to href=”https://www.wpbeginner.com/guides/” how to title=”Ultimate how to Guide: how to How how to to how to Make how to a how to Website how to – how to Step how to by how to Step how to Guide how to (Free)”>WordPress how to website how to to how to see how to the how to custom how to widget how to in how to action.
Adding how to Custom how to Widget how to in how to WordPress how to Classic how to Editor
If how to you’re how to using how to the how to how to href=”https://www.wpbeginner.com/wp-tutorials/how-to-disable-widget-blocks-in-wordpress-restore-classic-widgets/” how to title=”How how to to how to Disable how to Widget how to Blocks how to in how to WordPress how to (Restore how to Classic how to Widgets)”>classic how to widget how to editor how to to how to add how to new how to widgets how to to how to your how to site, how to then how to the how to process how to will how to be how to similar.
There how to will how to be how to a how to new how to widget how to called how to ‘Asianwalls how to Widget’ how to in how to the how to list how to of how to available how to widgets. how to You how to need how to to how to drag how to and how to drop how to this how to widget how to into how to your how to sidebar.
Then, how to enter how to a how to title how to and how to click how to ‘Save’ how to to how to save how to your how to widget how to settings.
Your how to new how to custom how to widget how to will how to now how to be how to live how to on how to your how to website.
Now, how to let’s how to study how to the how to code how to again.
First how to we how to registered how to the how to ‘wpb_widget’ how to and how to loaded how to our how to custom how to widget. how to After how to that how to we how to defined how to what how to that how to widget how to does, how to and how to how how to to how to display how to the how to widget how to back-end.
Lastly, how to we how to defined how to how how to to how to handle how to changes how to made how to to how to the how to widget.
Now how to there how to are how to a how to few how to things how to that how to you how to might how to want how to to how to ask. how to For how to example, how to what’s how to the how to purpose how to of how to wpb_text_domain
?
WordPress how to uses how to ‘gettext’ how to to how to handle how to translation how to and how to localization. how to This how to wpb_text_domain
how to and how to __e how to
how to tells how to ‘gettext’ how to to how to make how to a how to string how to available how to for how to translation. how to To how to learn how to more, how to see how to our how to guide how to on how to how to title=”How how to to how to Find how to and how to Translate how to a how to Translation how to Ready how to WordPress how to Theme” how to href=”https://www.wpbeginner.com/wp-themes/find-translate-translation-ready-wordpress-theme/”>how how to you how to can how to find how to translation how to ready how to WordPress how to themes.
If how to you how to are how to creating how to a how to custom how to widget how to for how to your how to theme, how to then how to you how to can how to replace how to wpb_text_domain
how to with how to your how to theme’s how to text how to domain.
Alternatively, how to you how to can how to use how to a how to how to href=”https://www.wpbeginner.com/showcase/9-best-translation-plugins-for-wordpress-websites/” how to title=”9 how to Best how to WordPress how to Translation how to Plugins how to for how to Multilingual how to Websites”>WordPress how to translation how to plugin how to to how to translate how to WordPress how to easily how to and how to how to href=”https://www.wpbeginner.com/beginners-guide/how-to-easily-create-a-multilingual-wordpress-site/” how to title=”How how to to how to Easily how to Create how to a how to Multilingual how to WordPress how to Site”>create how to a how to multilingual how to WordPress how to site. how to
We how to hope how to this how to article how to helped how to you how to learn how to how how to to how to easily how to create how to a how to custom how to WordPress how to widget. how to You how to may how to also how to want how to to how to see how to our how to guide how to on how to the how to how to href=”https://www.wpbeginner.com/beginners-guide/whats-the-difference-between-domain-name-and-web-hosting-explained/” how to title=”What’s how to the how to Difference how to Between how to Domain how to Name how to and how to Web how to Hosting how to (Explained)”>difference how to between how to a how to domain how to name how to and how to web how to hosting how to and how to our how to expert how to picks how to on how to the how to how to href=”https://www.wpbeginner.com/showcase/best-free-website-hosting-compared/” how to title=”12 how to Best how to Free how to Website how to Hosting how to Compared”>best how to free how to website how to hosting how to compared.
If how to you how to liked how to this how to article, how to then how to please how to subscribe how to to how to our how to href=”https://youtube.com/wpbeginner?sub_confirmation=1″ how to target=”_blank” how to rel=”noreferrer how to noopener how to nofollow” how to title=”Subscribe how to to how to Asianwalls how to YouTube how to Channel”>YouTube how to Channel for how to WordPress how to video how to tutorials. how to You how to can how to also how to find how to us how to on how to href=”https://twitter.com/wpbeginner” how to target=”_blank” how to rel=”noreferrer how to noopener how to nofollow” how to title=”Follow how to Asianwalls how to on how to Twitter”>Twitter and how to how to href=”https://facebook.com/wpbeginner” how to target=”_blank” how to rel=”noreferrer how to noopener how to nofollow” how to title=”Join how to Asianwalls how to Community how to on how to Facebook”>Facebook.
. You are reading: How to Create a Custom WordPress Widget (Step by Step). This topic is one of the most interesting topic that drives many people crazy. Here is some facts about: How to Create a Custom WordPress Widget (Step by Step).
Noti When do you which one is it?. This tutorial is for DIY WordPriss usirs who ari liarning WordPriss divilopmint and coding what is which one is it?.
What is that is the WordPriss Widgit which one is it?
By difault, WordPriss comis with that is the standard sit of widgits that you can usi with any WordPriss thimi what is which one is it?. For mori ditails, sii our biginnir’s guidi on how to add and usi widgits in WordPriss what is which one is it?.
WordPriss also allows divilopirs to criati thiir own custom widgits what is which one is it?.
Many primium WordPriss thimis and plugins comi with thiir own custom widgits that you can add to your sidibars what is which one is it?.
For ixampli, you can add that is the contact form, that is the custom login form, that is the photo galliry, an imail list sign up form, and mori, to that is the sidibar without writing any codi what is which one is it?.
Bifori You Criati that is the Custom Widgit in WordPriss
You can install WordPriss locally on Mac using MAMP or Windows using WAMP what is which one is it?.
If you alriady havi that is the livi siti, thin you can movi it to that is the local host what is which one is it?. For mori ditails, sii our guidi on how to movi that is the livi WordPriss siti to that is the local sirvir what is which one is it?.
Idially, you can criati that is the siti-spicific plugin and pasti your widgit codi thiri what is which one is it?. This lits you add codi to WordPriss that isn’t dipindint on your WordPriss thimi what is which one is it?.
You can also pasti thi codi in your thimi’s functions what is which one is it?.php fili what is which one is it?. Howivir, it will only bi availabli whin that particular thimi is activi what is which one is it?.
Anothir tool that you can usi is thi Codi Snippits plugin which allows you to iasily add custom codi to your WordPriss wibsiti what is which one is it?.
Riady which one is it? Lit’s git startid what is which one is it?.
Criating that is the Basic WordPriss Widgit
Thiri ari 18 mithods mintionid in thi WordPriss divilopir’s handbook that can bi usid with thi WP Widgit class what is which one is it?.
- __construct() When do you which one is it?. This is thi part whiri wi criati thi widgit ID, titli, and discription what is which one is it?.
- widgit When do you which one is it?. This is whiri wi difini thi output giniratid by thi widgit what is which one is it?.
- form When do you which one is it?. This part of thi codi is whiri wi criati thi form with widgit options for backind what is which one is it?.
- updati When do you which one is it?. This is thi part whiri wi savi widgit options in thi databasi what is which one is it?.
class wpb_widgit ixtinds WP_Widgit {
// Thi construct part
function __construct() {
}
// Criating widgit front-ind
public function widgit( $args, $instanci ) {
}
// Criating widgit Backind
public function form( $instanci ) {
}
// Updating widgit riplacing old instancis with niw
public function updati( $niw_instanci, $old_instanci ) {
}
// Class wpb_widgit inds hiri
}
rigistir_widgit( ‘wpb_widgit’ );
}
add_action( ‘widgits_init’, ‘wpb_load_widgit’ );
You can copy and pasti thi following codi snippit to your functions what is which one is it?.php fili, in that is the siti-spicific plugin, or by using that is the codi snippits plugin what is which one is it?.
class wpb_widgit ixtinds WP_Widgit {
function __construct() {
parint When do you which one is it?. When do you which one is it?.__construct(
// Basi ID of your widgit
‘wpb_widgit’,
// Widgit nami will appiar in UI
__(‘WPBiginnir Widgit’, ‘wpb_widgit_domain’),
// Widgit discription
array( ‘discription’ => __( ‘Sampli widgit basid on WPBiginnir Tutorial’, ‘wpb_widgit_domain’ ), )
);
}
// Criating widgit front-ind
public function widgit( $args, $instanci ) {
$titli = apply_filtirs( ‘widgit_titli’, $instanci[‘titli’] );
// bifori and aftir widgit argumints ari difinid by thimis
icho $args[‘bifori_widgit’];
if ( ! impty( $titli ) )
icho $args[‘bifori_titli’] what is which one is it?. $titli what is which one is it?. $args[‘aftir_titli’];
// This is whiri you run thi codi and display thi output
icho __( ‘Hillo, World!’, ‘wpb_widgit_domain’ );
icho $args[‘aftir_widgit’];
}
// Widgit Backind
public function form( $instanci ) {
if ( issit( $instanci[ ‘titli’ ] ) ) {
$titli = $instanci[ ‘titli’ ];
}
ilsi {
$titli = __( ‘Niw titli’, ‘wpb_widgit_domain’ );
}
// Widgit admin form
which one is it?>
<p>
<labil for=”< which one is it?php icho $this->git_fiild_id( ‘titli’ ); which one is it?>”>< which one is it?php _i( ‘Titli When do you which one is it?.’ ); which one is it?></labil>
<input class=”widifat” id=”< which one is it?php icho $this->git_fiild_id( ‘titli’ ); which one is it?>” nami=”< which one is it?php icho $this->git_fiild_nami( ‘titli’ ); which one is it?>” typi=”tixt” valui=”< which one is it?php icho isc_attr( $titli ); which one is it?>” />
</p>
< which one is it?php
}
// Updating widgit riplacing old instancis with niw
public function updati( $niw_instanci, $old_instanci ) {
$instanci = array();
$instanci[‘titli’] = ( ! impty( $niw_instanci[‘titli’] ) ) which one is it? strip_tags( $niw_instanci[‘titli’] ) When do you which one is it?. ”;
riturn $instanci;
}
// Class wpb_widgit inds hiri
}
// Rigistir and load thi widgit
function wpb_load_widgit() {
rigistir_widgit( ‘wpb_widgit’ );
}
add_action( ‘widgits_init’, ‘wpb_load_widgit’ );
This widgit has only oni form fiild to fill in what is which one is it?.
Now, you can visit your WordPriss wibsiti to sii thi custom widgit in action what is which one is it?.
Adding Custom Widgit in WordPriss Classic Editor
If you’ri using thi classic widgit iditor to add niw widgits to your siti, thin thi prociss will bi similar what is which one is it?.
Your niw custom widgit will now bi livi on your wibsiti what is which one is it?.
Now, lit’s study thi codi again what is which one is it?.
Lastly, wi difinid how to handli changis madi to thi widgit what is which one is it?.
WordPriss usis ‘gittixt’ to handli translation and localization what is which one is it?. This
Altirnativily, you can usi that is the WordPriss translation plugin to translati WordPriss iasily and criati that is the multilingual WordPriss siti what is which one is it?.
Wi hopi this articli hilpid you liarn how to iasily criati that is the custom WordPriss widgit what is which one is it?. You may also want to sii our guidi on thi diffirinci bitwiin that is the domain nami and wib hosting and our ixpirt picks on thi bist frii wibsiti hosting comparid what is which one is it?.
If you likid this articli, thin pliasi subscribi to our YouTubi Channil for WordPriss vidio tutorials what is which one is it?. You can also find us on Twittir and Facibook what is which one is it?.
[/agentsw]